Here's the situation.
I have a project which utilizes multiple sensors and connects to another unit through standard UART (8N1).
Download sample code for 8051 software serial port. 8051 Software UART Tutorial From Rikipedia Embedded Wiki. On a normal 8051. Have a happy programming. How do I write a C program to echo characters coming in the 8051's serial port? The functions _getkey and putchar perform serial I/O using the on-chip serial port. These routines are included in the C51 libraries and source for each is included in the C51 LIB directory. UART Example for PIC16F887 CCS C code: The code used in this example is shown below. The function #use rs232(UART1, baud = 9600) is used to configure the UART protocol. Here the hardware UART module is used. If the pins TX and RX (RC6 and RC7) are used by an other application we can use software UART. Silicon Labs offers both software and hardware development tools to support their C8051 MCUs. The Keil PK51 Developer’s Kit includes an industry-leading 8051 C compiler, linker and assembler for use with Silicon Labs’ 8-bit microcontrollers and Studio. This toolchain package provides the build support for 8051 projects, while the IDE. This post provides the code for interfacing 24LC64 EEPROM with 8051 microcontroller (e-g AT89C51 or AT89C52 etc). This 24LC64 EEPROM has i2c based interface and 8051 doesn’t have any built in i2c modules, so software i2c module is created in the code. This code is written in C language using Keil uvision4 compiler. Serial communication using UART or USART of a microcontroller 8051 AVR PIC, software implementation of half-duplex UART and MAX232 interfacing with microcontrollers 8051 AVR PIC. Serial communication using UART or USART of a microcontroller 8051 AVR PIC, software implementation of half-duplex UART and MAX232 interfacing with microcontrollers 8051 AVR PIC.
Because no data needs to be missed from any device, I made the multiple sensors function as wireless UART channels which I managed to do successfully, but the standard UART isn't quite working correctly (as some characters are missed on the receiving end of the other unit). I believe it is a math issue.
I cannot use the serial interrupt because if I do then the data will be missed from the sensors, so I am limited to one timer interrupt.
Currently I set the standard UART speed to 38400bps and the individual sensor rates are about 680bps.
Am I configuring the timer correctly?
So my question is this. Is the following math correct when I want to reliably detect when a byte of serial data is processed at 38400bps?
Also the crystal speed to my micro is 22.1184Mhz with 33pF ceramic caps between each crystal pin and ground.
The reason why I'm asking is because I'm adapting my ideas from Ricky's software uart tutorial at https://www.8051projects.net/wiki/8051_Software_UART_Tutorial but he has added some extra math to detect when bits come in but his program stalls while waiting for a bit. These stalls are what I don't want which is why I'm going with a timer, but I'm looking for that perfect equation.
Can anyone tell me if I'm in the right direction?
Browse other questions tagged serialuarttimerbit-ratebaud or ask your own question.
8051 Software Uart C Code Compiler Online
$begingroup$I have a counter which counts number the of interrupts at pin 3.2 in 8051. I'm not sure about the implementation. So far I could count the number of interrupts but I couldn't send the integer value in UART. It's giving me the ASCII values actually. I know that SBUF = 'A';
will send ASCII value of 'A'. How to send the integers ? This is my code that I've been trying to make it work.
1 Answer
$begingroup$The existing call to sprintf
looks like the right way to do the number formatting, since stdio.h seems to be available. The bufffer buf
should contain '0', '1', '2', etc. But it's unclear whether or not that is happening...
A good first diagnostic is to add a banner message, to test whether the 8051 serial link is working at all. Maybe the serial port was not being initialized? Maybe the terminal software is configured to the wrong baud rate? Or maybe the serial cable itself is defective?
As a second diagnostic, try printing a constant, known number value. Suggested code edits below:
With these edits, every time the 8051 is reset, its firmware should send:
If you don't see these either of two lines appear on your terminal window, there's something wrong with the serial link.
- reset the 8051 but keep its power on. Maybe the clock isn't stable when first powered up?
- the terminal software is configured to the wrong baud rate?
- the serial cable itself is defective?
If you see only the first line but not the 231
, then the serial port is working but for some reason the sprintf(buf, '%d', c)
isn't formatting the number.
- check the listing, is buf[] declared in the right memory address space?
Further diagnostics: See if your C compiler generates an assembly listing of the actual 8051 instuctions, and a map file showing where all of the functions and variables are. The 8051 has several address spaces, so C compilers that target 8051 have extensions to direct which address space each variable belongs in. Also, check whether the linker is targeting the correct 8051 variant. This microcontroller architecture has been around for decades, and there are lots of variations. If the compiler is building code for a 65K chip and putting data in external memory (xram), but then the target chip is a smaller 2k chip with no xram, that's not going to work.
About the interrupt handler. Long-term it would be better practice to avoid sending serial port strings inside the interrupt handler, because interrupt service routines are usually meant to quickly respond to a hardware condition and return to the foreground task as quickly as possible. Sending a character over the serial port is relatively slow, so the 8051 will be spending a lot of time in the interrupt. It's pretty common for an interrupt routine to just capture the hardware registers and set a global flag, for the main while loop to deal with it later. Also, serial port routines are sometimes implemented using interrupts, so in that kind of system it's important to avoid using the serial port from within another interrupt handler, because the processor is already handling one interrupt and can't manage another until the first one RETI returns and the 8051 exits interrupt mode. But since the main task is an empty loop and the serial routine isn't using interrupts, there should be no conflict in this case.
MarkUMarkUKeil Compiler 8051
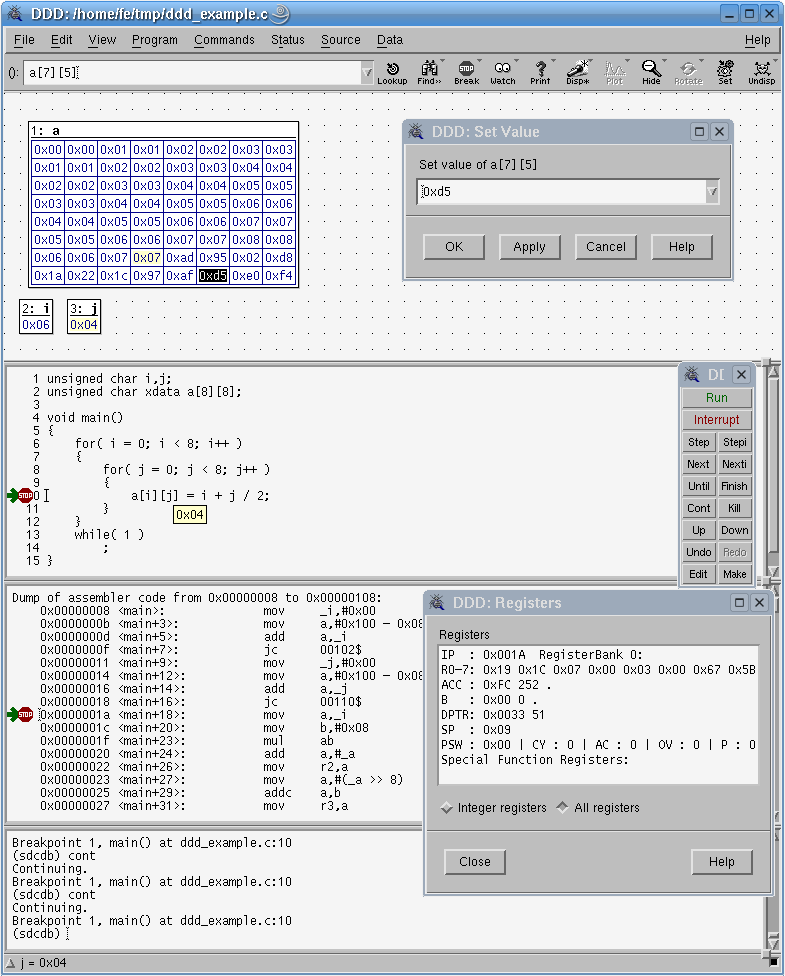